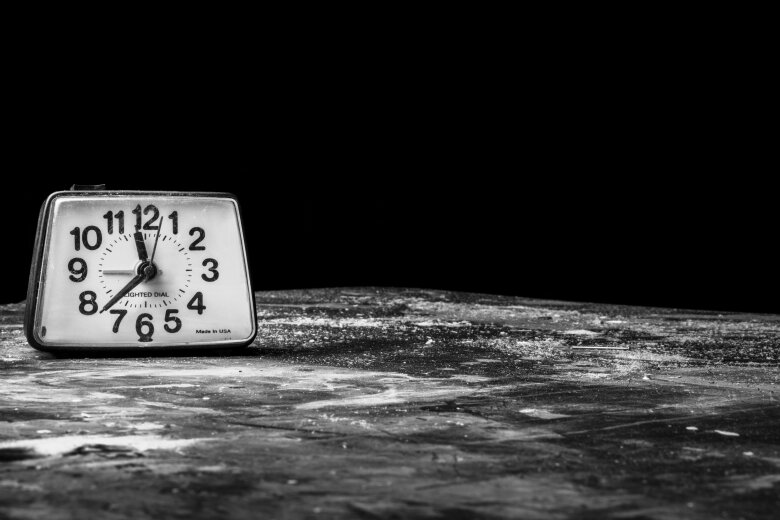
When I search and read code about time and date problems developers have, I noticed that a lot of people still use the old PHP functions like date()
, time()
or strtotime()
.
What about using the class DateTime instead?
DateTime
can do all the usual date and time operations you could ask for, and even more. Using DateTime
can save a lot of time when you have to do simple, or more complex operations on dates.
Now I see you asking: why using DateTime instead of a bunch of PHP date functions?
Here’s why:
DateTime
is definitely more robust to use. Less bug is always better.DateTime
is a class. You can use composition to modify his behavior easily. You need always the same date as input for your unit tests? Use your own instance ofDateTime
which always return the birthday of your dog!- Using one class is easier to understand for your fellow developer colleagues. Even if they don’t know
DateTime
, they just have to look to the documentation for one single class. It is definitely better than looking for a bunch of independent functions.
DateTime Instantiation and Formatting
The instantiation is easy: you can pass to the constructor a date correctly formatted, or nothing if you want to use the actual date and time.
Here the list of supported date and time formats you can inject in the constructor.
You can then format the date in order to display it, in two lines of code:
<?php
$dateTime = new DateTime('2016-01-01');
echo $dateTime->format('Y-m-d H:i:s');`
Output: 2016-01-01 00:00:00
<?php
$dateTime = new DateTime();
echo $dateTime->format('Y-m-d H:i:s');`
Output: whatever the current date is, correctly formatted.
Easy, isn’t it? You can as well precise the timezone you want as a second argument:
<?php
$date = new DateTime('2016-05-20', new DateTimeZone('Europe/Berlin'));
DateTime and Timestamps
You want to format an unreadable timestamp into a nice and shiny easy-to-read date?
<?php
$dateTime = new DateTime();
$dateTime->setTimestamp(1271802325);
echo $dateTime->format('Y-m-d H:i:s');
Output: 2010-04-20 22:25:25
Obviously you can as well output a timestamp if you need to:
<?php
$dateTime = new DateTime('2016-05-20');
echo $dateTime->getTimestamp();
Output: 1271802325
Adding or Subtracting Time
What about adding or retrieving a day, a minute, an hour of a date? You only need to use the proper formatting:
<?php
$dateTime = new DateTime('2016-01-01');
$dateTime->modify('+1 day');
echo $dateTime->format('Y-m-d H:i:s');`
Output: 2016-01-02 00:00:00
You can as well use the constructor if you work on the current date:
<?php
$dateTime = new DateTime('+1d');
echo $dateTime->format('Y-m-d H:i:s');`
Output the current date plus one day.
For the same result, you could as well instantiate the class DateInterval but you would have to use an interval specification which is way harder to read.
I never use this last solution personally, simply because I never need to.
Interval Between Different Dates
It’s very easy to use DateTime
classes to compare dates:
<?php
$datetime1 = new DateTime('2009-10-11 12:12:00');
$datetime2 = new DateTime('2009-10-13 10:12:00');
$interval = $datetime1->diff($datetime2);
echo $interval->format('%Y-%m-%d %H:%i:%s');
Output: 00-0-1 22:0:0
In this example we created two DateTime
objects. They will receive two different dates in their constructors.
In order to compare those two dates we use the method diff()
of the first DateTime
object with the second DateTime
object as argument.
The diff()
method will return a new object of type DateInterval
. If you want to format the difference between the two dates, you can use as well the format()
method of the class DateInterval
but be careful: it won’t accept the same formatting as the DateTime::format()
method.
The output indicate that there is 1 day and 22 hours difference between the two dates.
If you only want the seconds, hours or day of interval you can write:
<?php
$datetime1 = new DateTime('2009-10-11 12:12:00');
$datetime2 = new DateTime('2009-10-13 10:12:00');
$interval = $datetime1->diff($datetime2);
echo $interval->s;
echo $interval->h;
echo $interval->d;
Output:
0
22
1
Comparing DateTimes
If you need to compare two instances of DateTime, it’s as simple as writing:
<?php
$datetime1 = new DateTime('2009-10-11 12:12:00');
$datetime2 = new DateTime('2009-10-13 10:12:00');
if ($datetime1 > $datetime2) {
echo 'datetime1 greater than datetime2';
}
if ($datetime1 < $datetime2) {
echo 'datetime1 lesser than datetime2';
}
if ($datetime1 == $datetime2) {
echo 'datetime2 is equal than datetime1';
}
Output: datetime1 lesser than datetime2
Keep in mind that you need PHP 5.2.2 or greater for the comparisons to work. I really hope it’s the case!
You’re Now a Master of DateTime!
I invite you to read the PHP documentation about the class DateTime. You will never have to mess around with multiple functions and you will simplify your code a big time. Your colleague will praise you and your skills till the end of time.
Glory and fame will be at your door!
If you know other tricks and techniques with DateTime
, feel free to share your knowledge in the comment section.